The development of Robotics Software is a combersome task, often requiring the programming of multiple components for handling different sensors and actuators. Fortunately the adoption of software frameworks, namely the Robotic Operating System (ROS), has enabled the sharing of these components amongst practitioners, resulting in quicker prototpyings. Nonetheless, ROS is a complex framework, with a steep learning curve, requiring the understanding of the framework achitecture, messaging system, multiple programming languages, highly tied the operating system, etc. while, not supporting out-of-the-box more sophisticated simulators, such as MuJoCo – of high interest to current Machine Learning/Robotics research. On the other hand, MuJoCo, which can be set-up and run from a single Python script, does not include many capabilities for modularity or integration with real world hardware. To address this gap, we introduce the Yarok framework. Using Yarok practitioners can describe components using an slightly extended MuJoCo markup language, that are directly represented as Python classes, and have full experimental setups in a few clear lines of code. Further, the low level interfaces, for each component, for interacting with the environment (MuJoCo, or real world), can be also written as Python classes, and are enabled by the framework, for the corresponding environment.
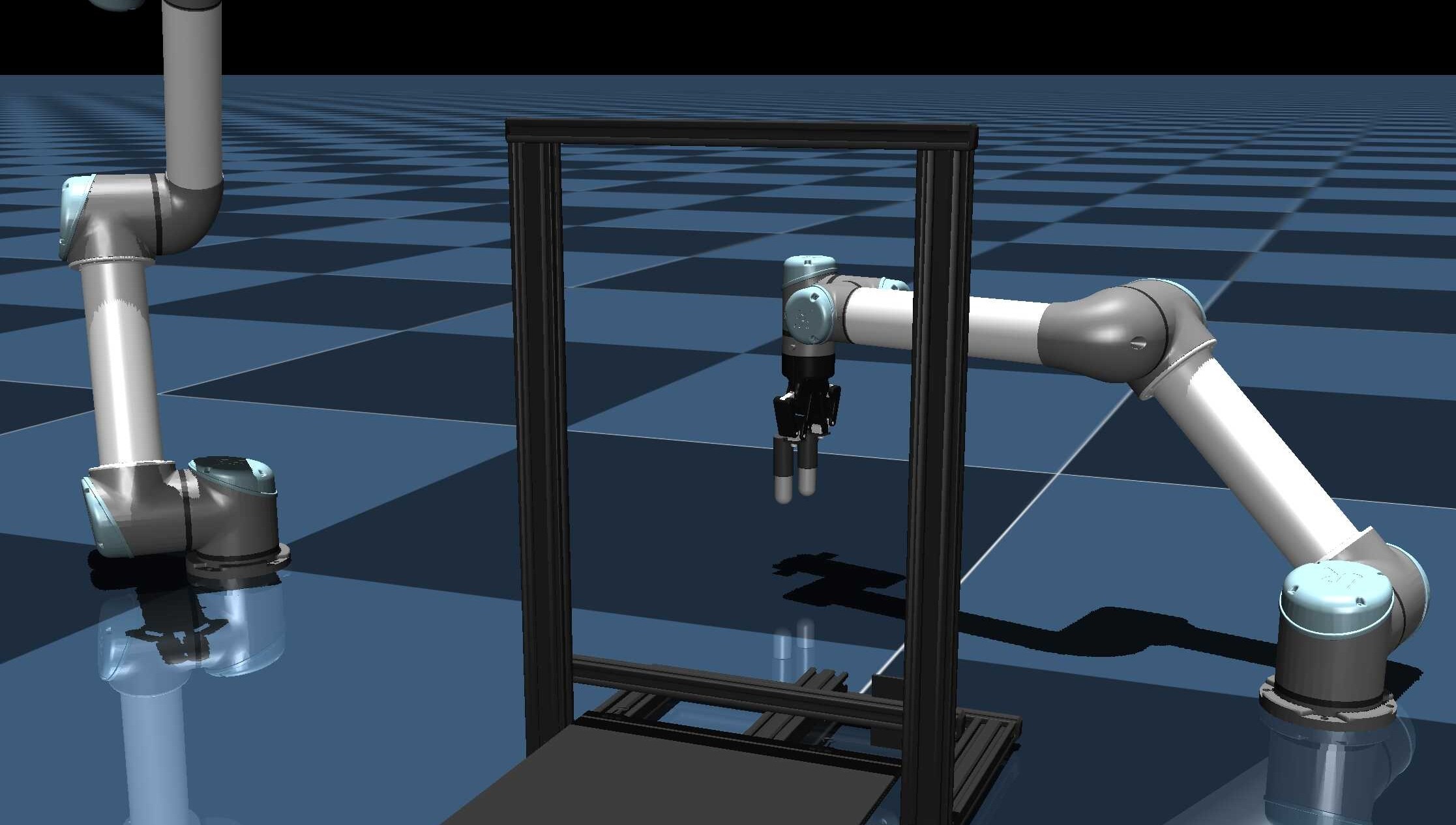
The Component
The central concept in Yarok is the component. A Component is a Python annotated class that allows you to bundle together the component controller and template description (using extended MuJoCo MJCF). In the component, you can also define environment interfaces that, at runtime, depending on what enviroment yarok is running, override the environment-independent controller class.
1 |
Composing components
Yarok allows you to easily compose complex robots using components of sensors and actuators. Bellow, we show how o include a custom gripper component onto your robot model, as well as nesting a sensor onto the gripper finger. Further, you’re able to request the corresponding gripper and sensor instance controllers to be injected onto the robot controller.
1 | from my_shared_components import MyGripper, MyTouchSensor |
Dynamic templates
Similarly to xacro in the Robotic Operating System (ROS), Yarok adds config variables and if/for statements to templates. See below how we defined a parameterizable tumble tower of blocks using these macros. Define the variables in the defaults section, and override it when running the platform.
1 | <for each='range(rows)' as='z'> |
MJC Interface
1 | from yarok import interface |
Running your environment
Once you have your components tree defined, you just wrap it onto a Platform object and run it. By default, Yarok runs on the simulation environment, loading MuJoCo interfaces, but you can also configure it to run on “real” environment, and it will load the real interfaces. This way, you can write your behaviours once and run them both in simulation and the real world.
1 | from yarok import Platform, PlatformMJC |
Example
1 | <body euler="0 0 1.57" pos="-0.15 0.4 0.3"> |
In this post we have just give you a quick glance at what you can do with Yarok, if you would like to learn more and getting started using it, visit the project repository and start experimenting.